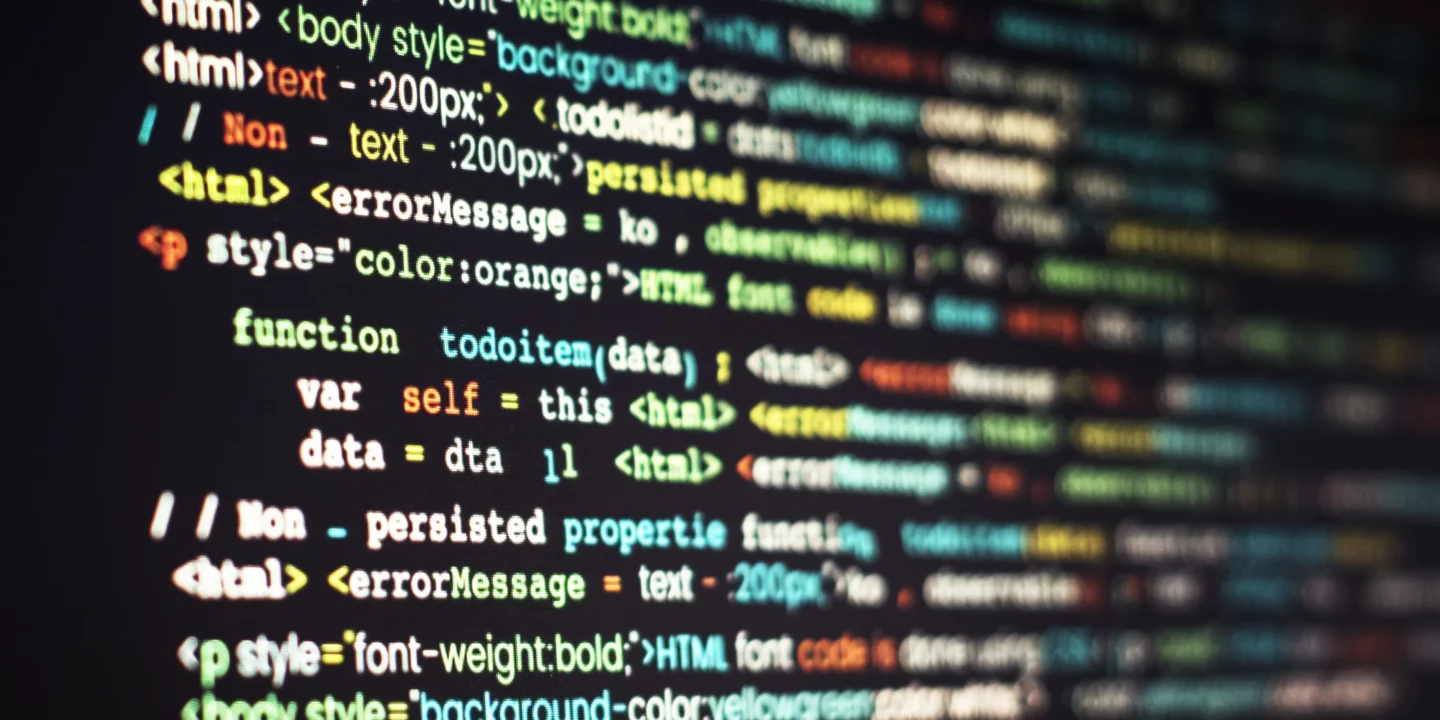
Table of Contents
In this article you will get to know all about meta programming, how it is making a great change in programming, what it is, its use, and how smartly you can do programming with the approach of meta programming.
What is Meta in programming?
Meta in programming is the technique that deals with data; it can generate code automatically and can modify the structure of code at a high level. It makes the programming more flexible and efficient.
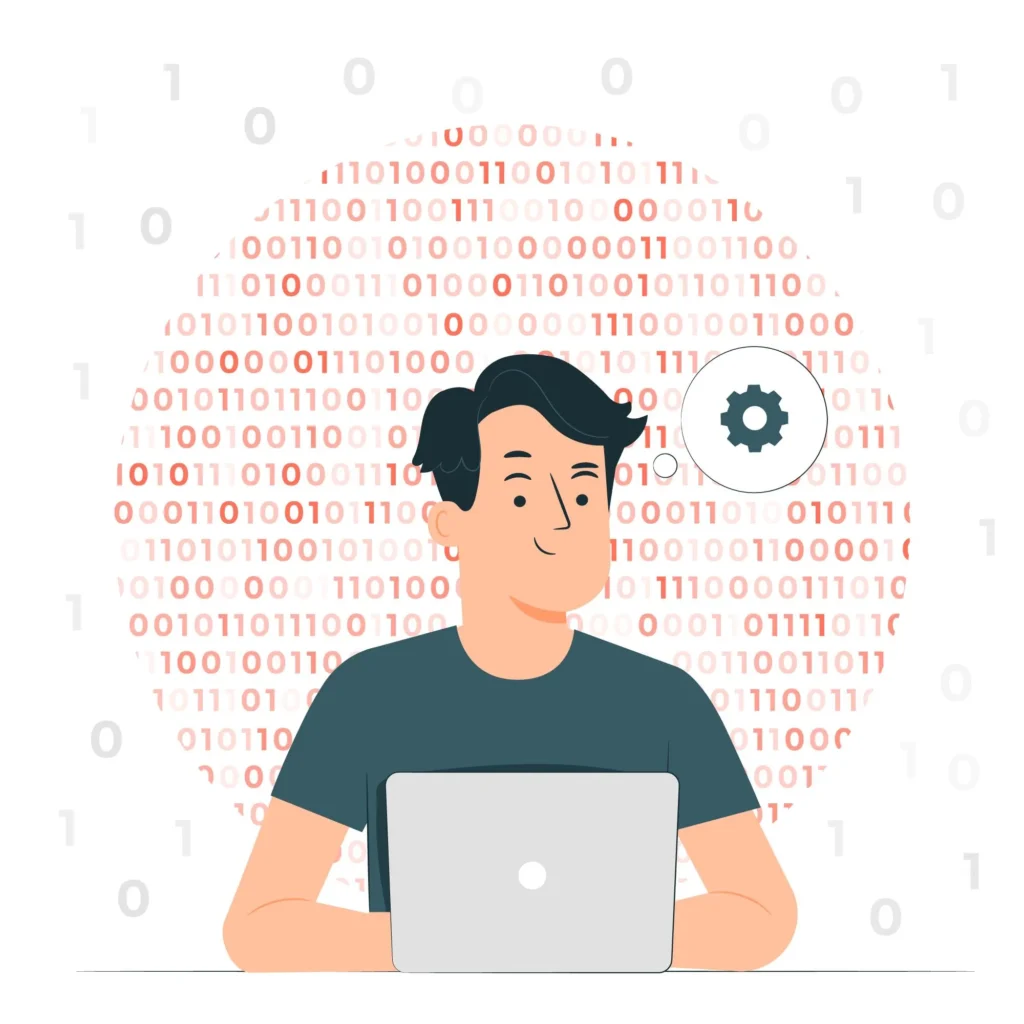
According to https://devopedia.org/
“Metaprogramming can be described as the technique of specifying generic software source templates from which classes of software components, or parts thereof, can be automatically instantiated to produce new software components.”
Following are the examples of metaprogramming in different computer languages.
Metaprogramming in python
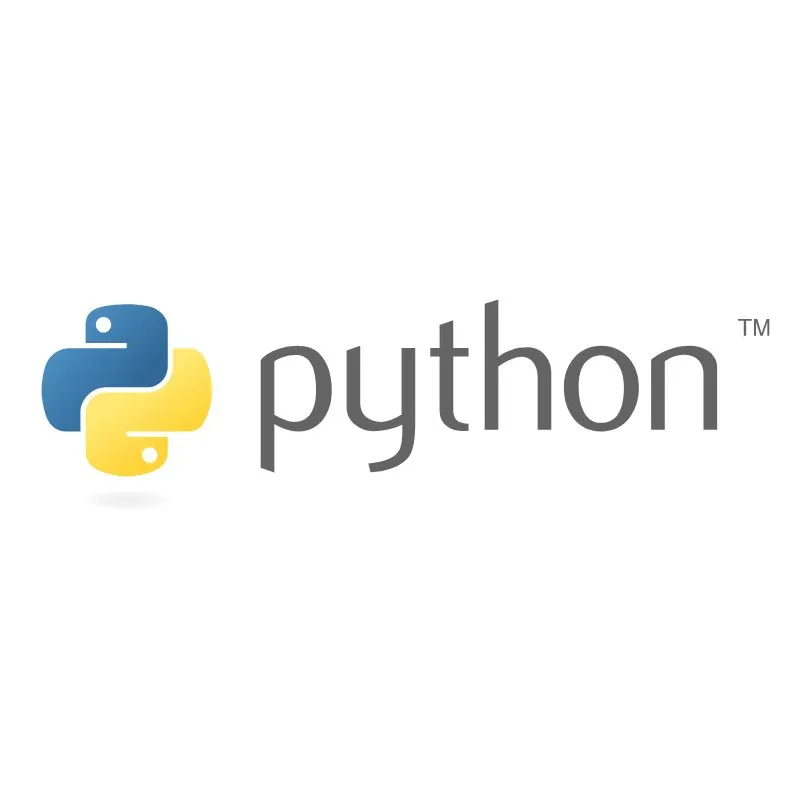
Decorators in Python
In Python, decorators are wrappers for functions. By using decorators, you can add new features to the code without disturbing its original logic. It makes code easy to read, and if any functionality needs to be deleted or modified, decorators allow only that particular functionality to be worked on.
Let’s understand it by a coding example in python:
def my_decorator(func):
def wrapper():
print("before the function is called.")
func()
print("after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Now the question arises: where is metaprogramming in Python decorators? So, as discussed, metaprogramming allows us to dynamically modify the functions’ behavior. In this piece of code, my_decorator had wrapped the function say_hello and modified its code like that.
Metaclasses in python
Classes define the behavior of objects, while metaclasses define the behavior of classes.
Let’s understand with a code that it is an example of metaprogramming.
# Define a metaclass
class MyMeta(type):
def _new_(cls, name, bases, dct):
print(f"Creating class: {name}")
dct['custom_attr'] = "Added by metaclass"
return super()._new_(cls, name, bases, dct)
# Use the metaclass to create a class
class MyClass(metaclass=MyMeta):
pass
# Access the dynamically added attribute
print(MyClass.custom_attr) # Output: Added by metaclass
In this example, MyMeta is the meta class and runs its method, which is _new_. This method can modify the class during the creation, so because of this, it’s an example of a metaclass, as it can make changes or modifications in the class during its creation without disturbing the whole code.
Metaprogramming in java
Just like in python there are many examples of meta programming in JAVA.
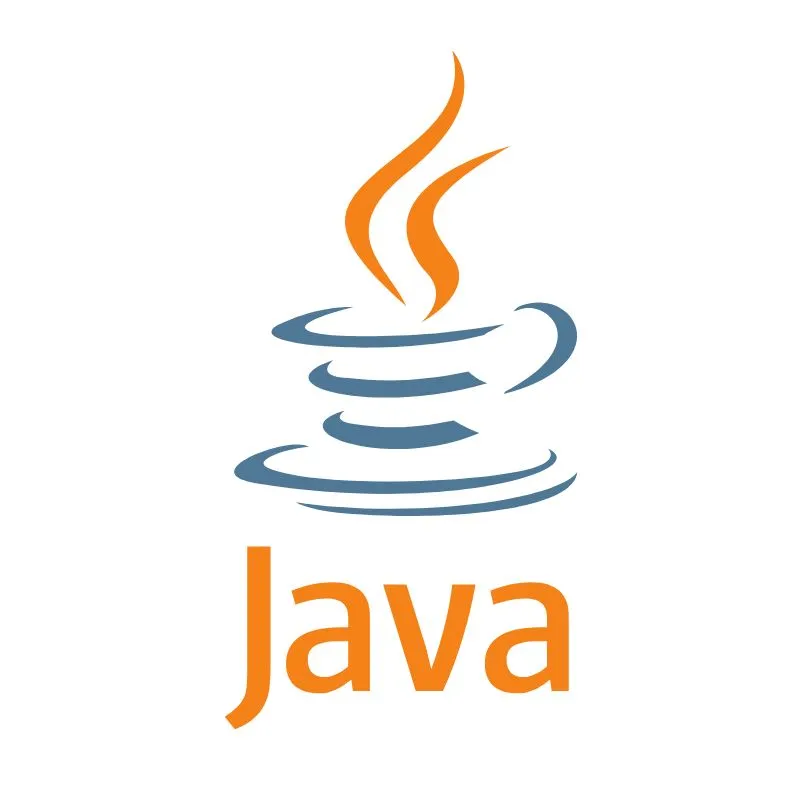
Reflection in JAVA
In Java reflection is used to manipulate the classes, methods, interfaces, and constructors at runtime if required. It can easily be done by calling methods or dynamically creating objects if you do not remember the name. Reflection in JAVA is useful if your code requires some addition in the future.
Let’s understand more by an example.
class Person {
public void greet() {
System.out.println("Hello!");
}
}
Now using Reflection to call the greet method dynamically
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) {
try {
// Get the Person class
Class<?> personClass = Class.forName("Person");
// Create an instance of Person
Object personInstance = personClass.newInstance();
// Get the greet method
Method greetMethod = personClass.getMethod("greet");
// Call the greet method using reflection
greetMethod.invoke(personInstance); // Output: Hello!
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, code is being inspected at the runtime, checks the class person and its methods, and calls the greet method.
Metaprogramming in Ruby
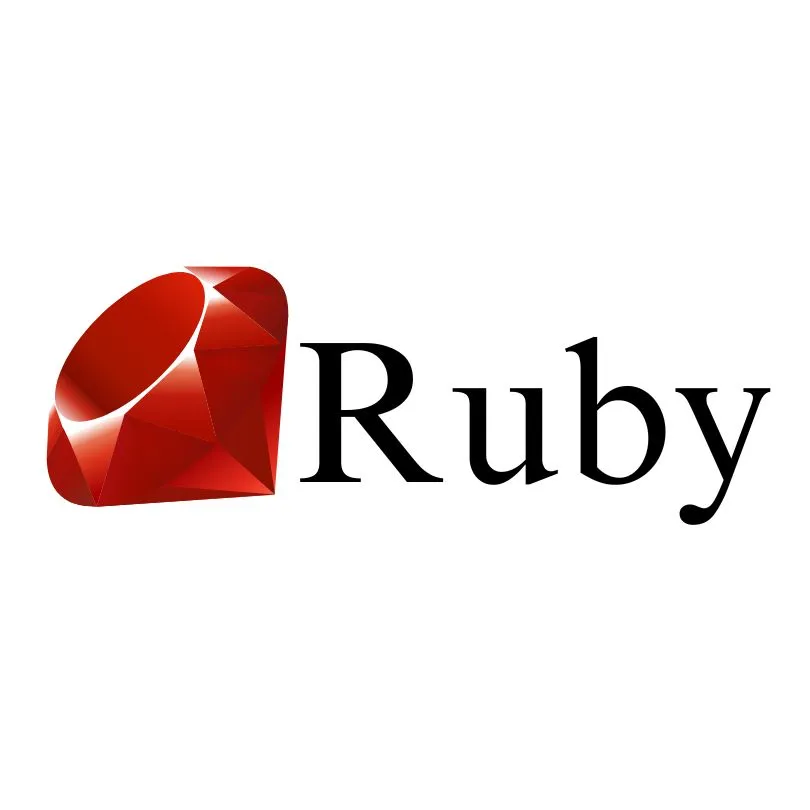
In Ruby, methods can be defined at runtime, and classes can be modified. By using method_missing, which is dynamically called under uncertain circumstances, like undefined methods dynamically. In Ruby, define_method is used to manipulate class behavior dynamically.
Let’s have a look at examples of it:
Example # 01
class Example
# Define a method dynamically
define_method(:greet) do
puts "Hello, Meta-programming in Ruby!"
end
end
obj = Example.new
obj.greet # Output: Hello, Meta-programming in Ruby!
In this example define_method had created method greet while the program was running.
Example # 02
Let’s have a look to another example which is for an Undefined method in ruby:
class DynamicClass
def method_missing(method_name, *args)
puts "You called #{method_name} with arguments: #{args.inspect}"
end
end
obj = DynamicClass.new
obj.say_hello("world") # Output: You called say_hello with arguments: ["world"]
Here method_missing calls an undefined method, handling it dynamically so it’s a good example for metaprogramming.
Metaprogramming in JavaScript
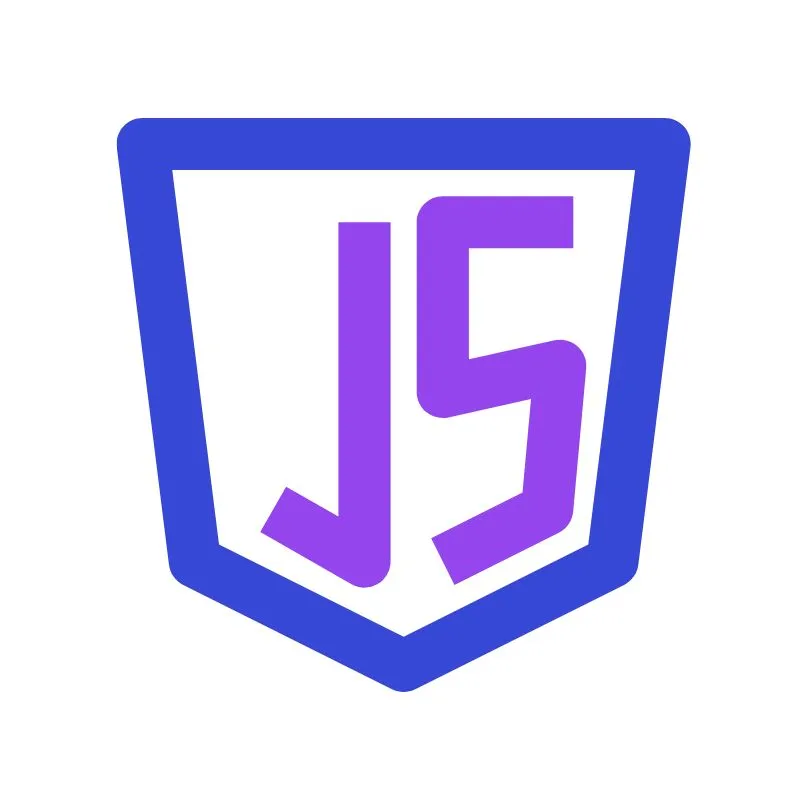
Just like in other programming languages, we can see the use of metaprogramming in JavaScript too; we can see its use to make code more flexible, reusable, and efficient. If you want to be a reliable programmer, then it’s necessary to make your code effective and neat.
In JavaScript we use a proxy, which acts as a bridging element between an object and code; it can customize the operations to another object and redefine operations like method calls in runtime, so it’s a great example of metaprogramming.
Let’s have a look to an example:
const user = { name: "Alice" };
const handler = {
get: (target, prop) => {
return prop in target ? target[prop] : "Property not found!";
}
};
const proxyUser = new Proxy(user, handler);
console.log(proxyUser.name); // Output: Alice
console.log(proxyUser.age); // Output: Property not found!
In this example proxy changes the behavior of property access, without changing the object user. It’s an example of metaprogramming.
Why is metaprogramming useful?
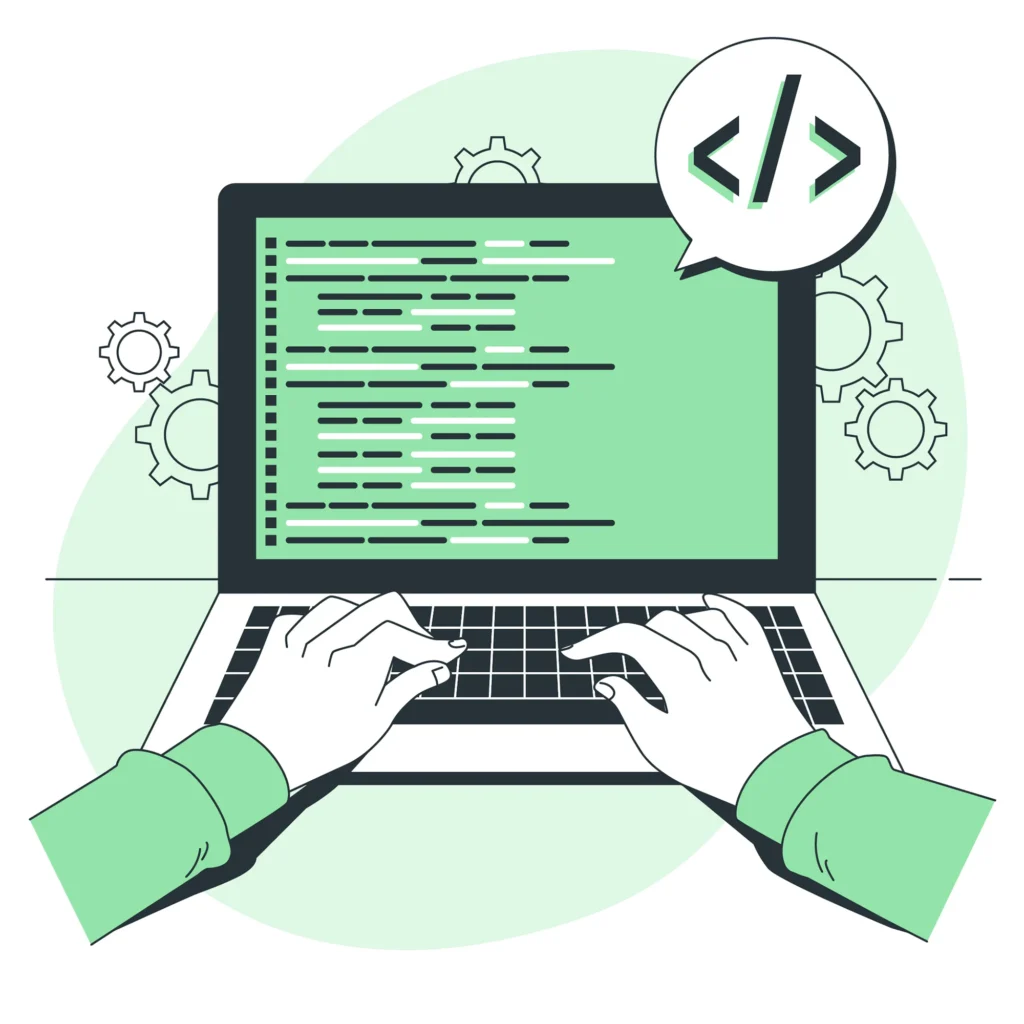
- Create classes and functions quickly for any purpose, at runtime at runtime dynamically.
- Using metaprogramming, code enhancement is easy, any new feature can always be defined in the future without affecting the rest of the code.
- Detailed procedures in systems and codes can be run by it.
- Further, the software architectures can be altered dynamically like the creation of GUI Interfaces.
- Metaprogramming is good for debugger and runtime information; it helps to notice problems on time.
Importance of metaprogramming in Web development
In web development, meta-programming is very useful; it saves time when automatically generating code. There’s a web framework like Ruby on Rails that generates the CRUD operations automatically against any new product model you add, without making it again and again separately for different product models and updating the database simultaneously.
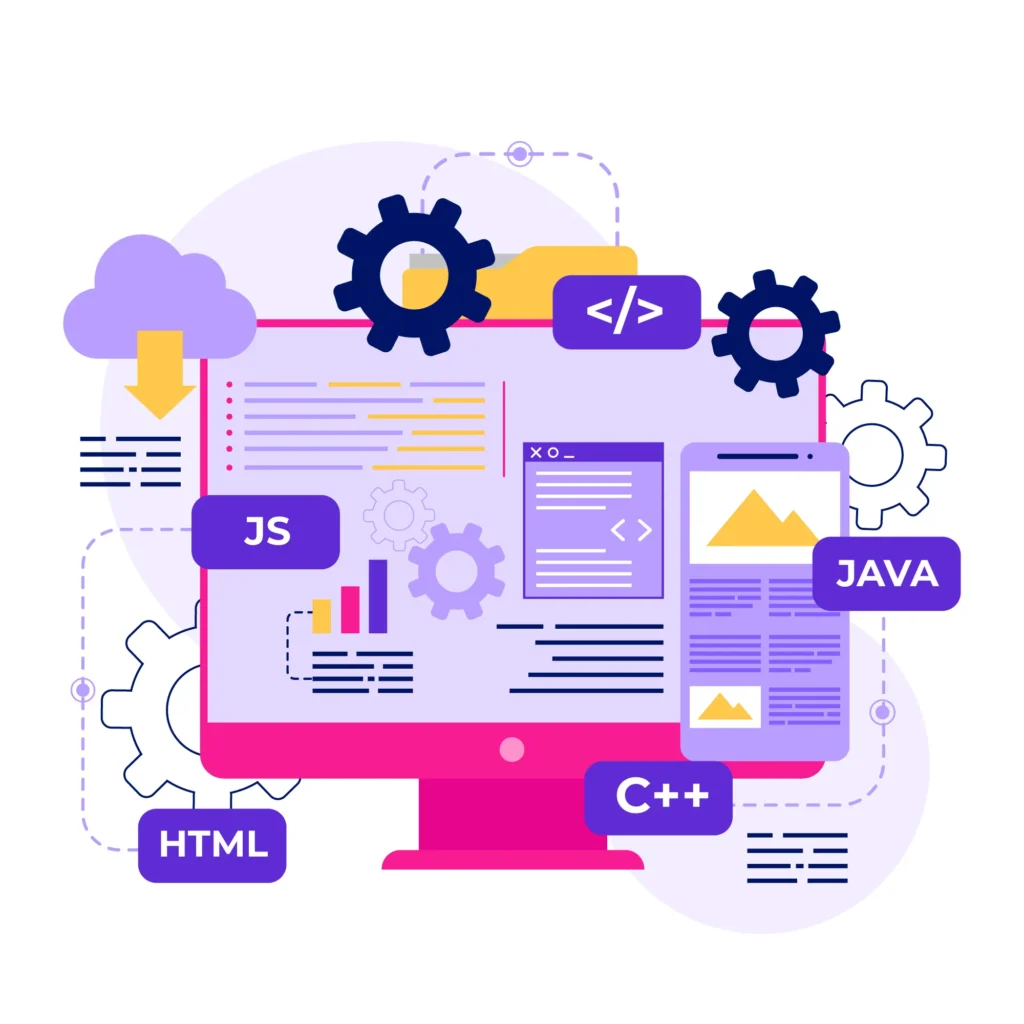
When using CMS like WordPress, it allows you to add plugins without changing the core code of the website; any feature can be added dynamically, and its code logic won’t get changed.
Conclusion
Metaprogramming makes the code smarter and more flexible allowing it to execute the act of adaptive code as well as new code. It can help to minimize the time after which a particular sequence is repeated, provide solutions to complex questions, and make the coding process much easier while at the same time minimizing repetitive coding, hence a lot of errors are avoided.
It enables one to develop progressive frameworks, build programs that address organizational-specific needs, and enhance performance. This is why metaprogramming will help you evolve your powers, optimize heavy processes, and expand the scope of opportunities in software development, making you a more valuable and creative developer.
No Comments